Table of Contents
Export current axis to file
A task both easy to perform and often asked for: Exporting the current display/axis to a figure file, including support for several file formats and geometries. The GUI elements for that could look like those displayed in Fig. 1 (actually, a real-world example from the trEPR GUI).
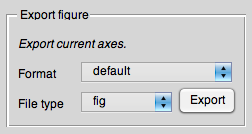
fig2file
routine and its configuration. There is only a bit of coding necessary to keep it as modular as possible.
Conveniently, the underlying functionality is completely covered by the fig2file
routine and its configuration. Therefore, there is only a bit of coding necessary to keep it as modular as possible. How to do that will be outlined below.
The GUI elements
defaultBackground = get(hMainFigure,'Color'); mainPanelWidth = 260; mainPanelHeight = 420; panel_size = 240; % ... ppX_pY = uipanel('Tag','display_panel_axesexport_panel',... 'Parent',pp2,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'Units','Pixels',... 'Position',[10 mainPanelHeight-280 panel_size 120],... 'Title','Export figure'... ); uicontrol('Tag','display_panel_axesexport_description',... 'Style','text',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'Units','Pixels',... 'HorizontalAlignment','Left',... 'FontAngle','oblique',... 'Position',[10 70 panel_size-20 20],... 'String','Export current axes.'... ); uicontrol('Tag','display_panel_axesexport_format_text',... 'Style','text',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'HorizontalAlignment','left',... 'Units','Pixels',... 'Position',[10 40 60 20],... 'String','Format'... ); hAxesExportFormat = uicontrol('Tag','display_panel_axesexport_format_popupmenu',... 'Style','popupmenu',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'Units','Pixels',... 'Position',[panel_size-170 45 160 20],... 'String','default|square|fullwidth|fullwidthhalfheight|halfwidth',... 'TooltipString','Select format of exported graphics'... ); uicontrol('Tag','display_panel_axesexport_filetype_text',... 'Style','text',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'HorizontalAlignment','left',... 'Units','Pixels',... 'Position',[10 10 60 20],... 'String','File type'... ); uicontrol('Tag','display_panel_axesexport_filetype_popupmenu',... 'Style','popupmenu',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'Units','Pixels',... 'Position',[panel_size-170 15 100 20],... 'String','fig|eps|pdf|png',... 'TooltipString','Select type of graphics file'... ); uicontrol('Tag','display_panel_axesexport_pushbutton',... 'Style','pushbutton',... 'Parent',ppX_pY,... 'BackgroundColor',defaultBackground,... 'FontUnit','Pixel','Fontsize',12,... 'Units','Pixels',... 'Position',[panel_size-70 10 60 30],... 'String','Export',... 'TooltipString','Export current axis to graphics file with given format',... 'Callback',{@pushbutton_Callback,'exportFigure'}... );
The Callback
Note that despite the two popup menus and the one pushbutton, only one callback, the one for the button, is needed. This is due to the fact that the button callback will get the current state of the menus and act accordingly.
The following listing is a real-world example that was tested and therefore should work, provided all the functions it relies on do exist on the Matlab™ path. It makes use of several design principles layed out elsewhere, such as generic callbacks with an “action” argument as third input argument, the use of try-catch
statements and excessive error handling via trEPRgui_bugreportwindow
.
function pushbutton_Callback(~,~,action) try if isempty(action) return; end % Get appdata and handles of main window mainWindow = trEPRguiGetWindowHandle(mfilename); ad = getappdata(mainWindow); gh = guihandles(mainWindow); % Make life easier active = ad.control.spectra.active; % Return immediately if there is no active dataset if isempty(active) || active == 0 return; end switch action case 'exportFigure' % Open new figure window and make it invisible newFig = figure('Visible','Off'); % Plot into new figure window updateAxes(newFig); % Get export format figExportFormats = cellstr(... get(gh.display_panel_axesexport_format_popupmenu,'String')); exportFormat = figExportFormats{... get(gh.display_panel_axesexport_format_popupmenu,'Value')}; % Get file type to save to fileTypes = cellstr(... get(gh.display_panel_axesexport_filetype_popupmenu,'String')); fileType = fileTypes{... get(gh.display_panel_axesexport_filetype_popupmenu,'Value')}; % Generate default file name if possible, be very defensive if ad.control.spectra.visible [~,fileNameSuggested,~] = ... fileparts(ad.data{active}.file.name); else fileNameSuggested = ''; end % Ask user for file name [fileName,pathName] = uiputfile(... sprintf('*.%s',fileType),... 'Get filename to export figure to',... [fileNameSuggested '-whateverSeemsSensible']); % If user aborts process, return if fileName == 0 close(newFig); return; end % Create filename with full path fileName = fullfile(pathName,fileName); % Save figure, depending on settings for file type and format status = fig2file(newFig,fileName,... 'fileType',fileType,'exportFormat',exportFormat); if status trEPRadd2status(status); end % Close figure window close(newFig); return; % Include here your other actions % case 'whatever' otherwise disp([mfilename ' : pushbutton_Callback() : '... 'Unknown action "' action '"']); return; end catch exception try msgStr = ['An exception occurred. '... 'The bug reporter should have been opened']; trEPRadd2status(msgStr); catch exception2 exception = addCause(exception2, exception); disp(msgStr); end try trEPRgui_bugreportwindow(exception); catch exception3 % If even displaying the bug report window fails... exception = addCause(exception3, exception); throw(exception); end end end
This code listing relies on a function updateAxes()
that can get a figure handle (that is: not an axis handle) to plot to. Furthermore, of course, it relies on fig2file
as the backend to save the current figure/axis to a file.
The other infrastructure
A piece of code that normally goes to the “Initialization tasks” of a GUI function file, but clearly below the definition of the actual GUI components, is the one that reads the export formats (aka geometries) from the fig2file ini file:
% Read configuration for export formats (geometries) from ini file exportFormatsConfigFile = fullfile(trEPRinfo('dir'),'figure','fig2file.ini'); exportFormats = trEPRiniFileRead(exportFormatsConfigFile); % Set export formats (geometries) set(hAxesExportFormat,'String',fieldnames(exportFormats));
To complete this example, the following is an excerpt from the updateAxes()
function that can get a figure handle as additional argument:
function updateAxes(varargin) try % ... % Get axis handle if nargin && ishandle(varargin{1}) mainAxes = newplot(varargin{1}); else mainAxes = gca; end % IMPORTANT: Set main axis to active axis axes(mainAxes); %#ok<MAXES> % ...